Create Fade In/Out Effects
This tutorial shows how to create fade in/out (scene transition) effects using TWEEN.
The fade in/out effect can be created by adjusting the opacity of a black image.
We will use TWEEN’s .onStart()
/ .onComplete()
/ .easing()
/ .yoyo()
/ .repeat()
methods.
Adding a Black Image to the GUI
Upload the provided black image asset and add it to the GUI.
Download Black Image
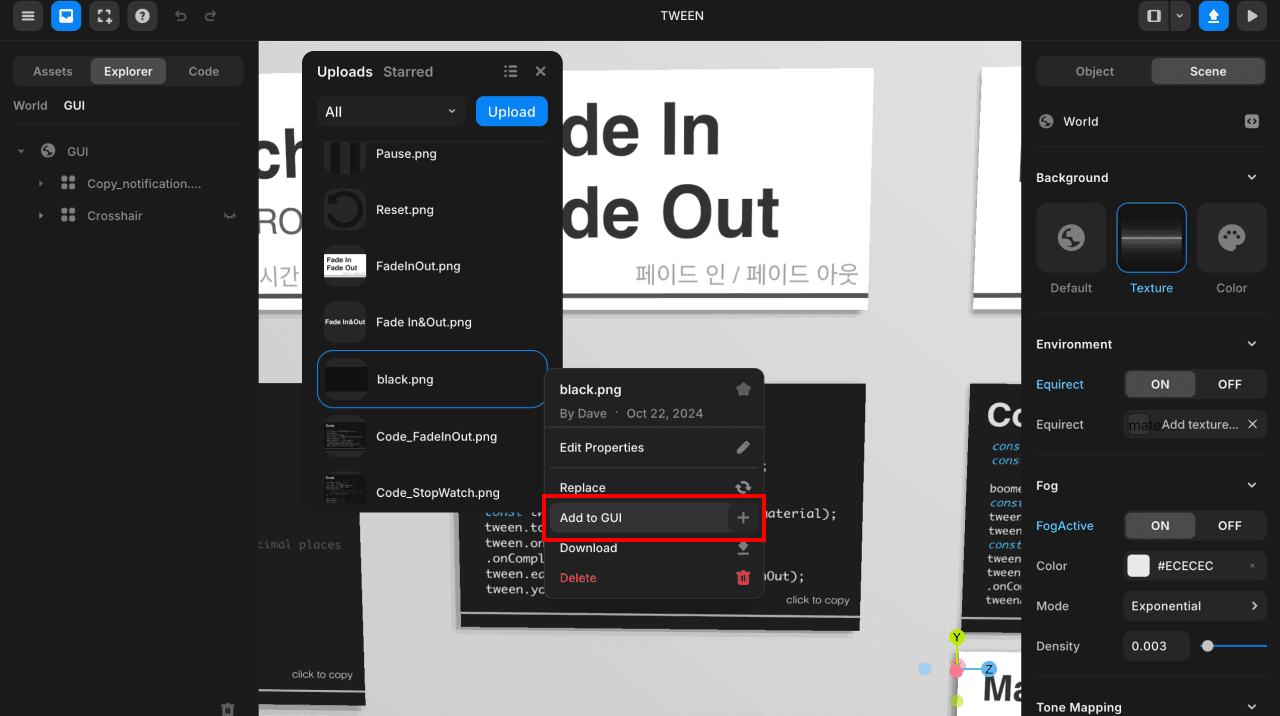
Basic Setup for the Black Image GUI
a. Set the object’s name to black.
b. Configure it to respond to screen size changes and cover the entire screen.
    b-1. Set the size units to percent (%) and set both x and y values to 100.
    b-2. Turn off Maintain AspectRatio to prevent the black image from keeping its ratio.
c. Set the transparecy to 100 to ensure the black image starts invisible.
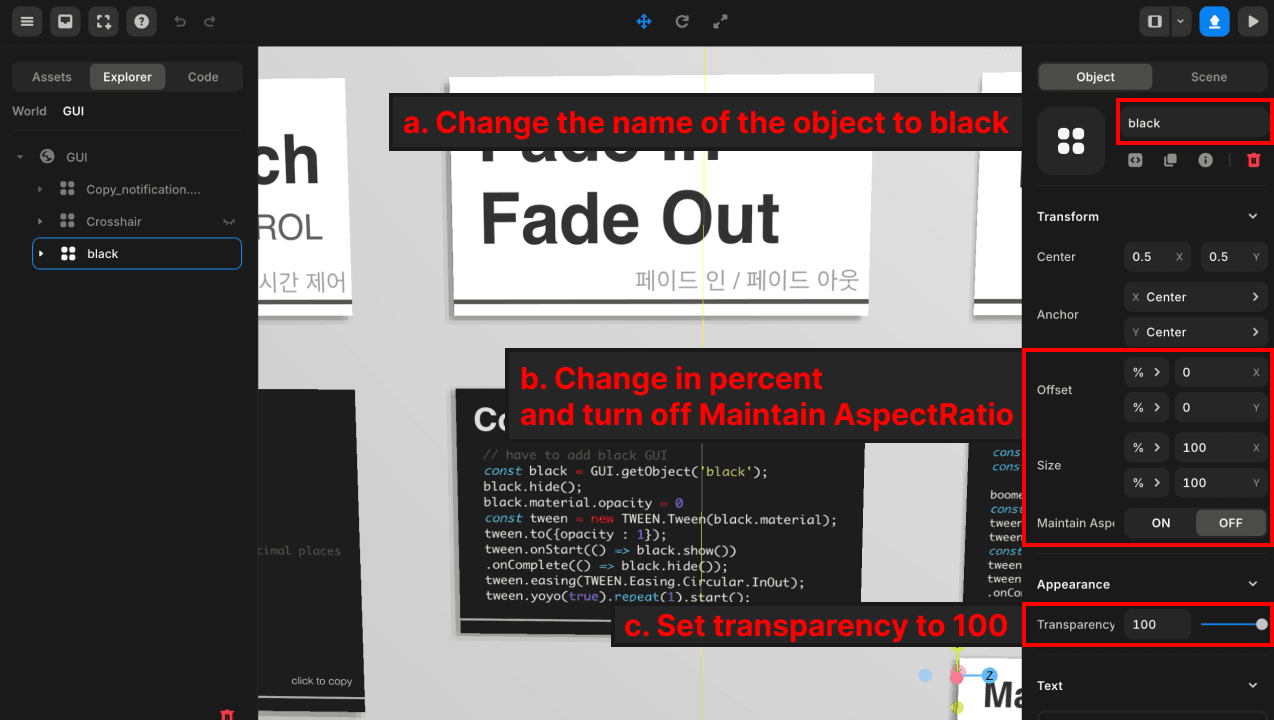
To create a responsive GUI, you must change the unit from px to % (percent).
Additionally, you can toggle Maintain AspectRatio ON/OFF based on whether you want to maintain the ratio.
Writing TWEEN Script for Fade In/Out
Add the object (client) script to the black image GUI. Use TWEEN to adjust the opacity of the black image.
Since we are using yoyo()
, the transition time for fade in/out will be double the duration
in tween.to(object, duration)
.
const black = GUI.getObject('black');
black.hide();
black.material.opacity = 0;
const tween = new TWEEN.Tween(black.material);
// Adjust the fade in/out transition time, the default is 1000ms
tween.to({opacity : 1});
tween.onStart(() => black.show())
.onComplete(() => black.hide());
tween.easing(TWEEN.Easing.Circular.InOut);
tween.yoyo(true).repeat(1);
The default transition time for TWEEN is 1000 milliseconds, or 1 second.
The black image is also a Sprite object in the world, which means it can be affected by raycasting, i.e., .onClick()
.
Therefore, use .onStart()
/ .onComplete()
to control when the image appears and disappears during the fade in/out.
Executing TWEEN During Screen Transitions
Move the player when the opacity of the black image reaches 1 (when the screen is completely covered).
tween.onUpdate(() => {
// Move the player when the black image's opacity is 1
if(black.material.opacity === 1) {
PLAYER.spawn();
}
});
function Update(dt) {
if(PLAYER.position.y < -5) tween.start();
}
When using .yoyo()
, no callback function is executed upon reaching the target value, so
you can handle the yoyo
start condition inside the .onUpdate()
method with a conditional statement.