Raycasting
The Raycaster is a tool used to check whether a ray emitted from a specific point in a 3D environment intersects with objects. It is primarily used to enable interactions based on user input.
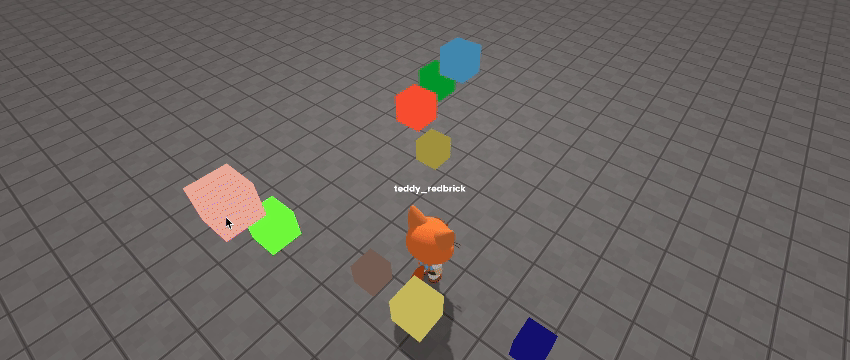
Steps
Initialize the Raycaster and Mouse Vector:
const raycaster = new THREE.Raycaster();
const mouse = new THREE.Vector2();
Normalize Mouse Coordinates:
When the mouse is clicked, normalize the mouse coordinates based on the size of the browser window.
function OnPointerDown(event) {
mouse.x = (event.clientX / window.innerWidth) * 2 - 1;
mouse.y = -(event.clientY / window.innerHeight) * 2 + 1;
}
Set Up the Raycaster
Set the raycaster based on the normalized mouse coordinates and the camera.
raycaster.setFromCamera(mouse, camera);
Handle Intersection Results
When an intersection is found, perform operations on the corresponding object. For example, this code changes the objectโs color.
const intersects = raycaster.intersectObjects(objects);
if (intersects.length > 0) {
intersects.forEach(intersect => {
intersect.object.material.color.set(Math.random() * 0xffffff); // ๋๋ค ์์์ผ๋ก ๋ณ๊ฒฝ
});
}
Complete Code
const avatar = REDBRICK.AvatarManager.createDefaultAvatar();
const camera = WORLD.getObject("MainCamera");
avatar.setFollowingCamera(camera);
avatar.setDefaultController();
const objects = []; // ์ฌ๋ฌ ๊ฐ์ ๊ฐ์ฒด๋ฅผ ์ ์ฅํ ๋ฐฐ์ด
const raycaster = new THREE.Raycaster();
const mouse = new THREE.Vector2();
// ๊ฐ์ฒด ์์ฑ ๋ฐ ๋ฐฐ์ด์ ์ถ๊ฐ
for (let i = 0; i < 10; i++) {
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
cube.position.set(Math.random() * 10 - 5, Math.random() * 10 - 5, Math.random() * 10 - 5);
WORLD.add(cube);
objects.push(cube);
}
// ๋ง์ฐ์ค ํด๋ฆญ ์ด๋ฒคํธ ํธ๋ค๋ฌ
function OnPointerDown(event) {
mouse.x = (event.clientX / window.innerWidth) * 2 - 1;
mouse.y = -(event.clientY / window.innerHeight) * 2 + 1;
raycaster.setFromCamera(mouse, camera);
const intersects = raycaster.intersectObjects(objects); // ์ฌ๋ฌ ๊ฐ์ฒด์ ๊ต์ฐจ ๊ฒ์ฌ
if (intersects.length > 0) {
intersects.forEach(intersect => {
intersect.object.material.color.set(Math.random() * 0xffffff); // ๋๋ค ์์์ผ๋ก ๋ณ๊ฒฝ
});
}
}
function Start() {
// ์ด๊ธฐํ ์์
(ํ์ ์)
}
function Update(dt) {
// ๋งค ํ๋ ์ ์
๋ฐ์ดํธ ์์
(ํ์ ์)
}