Creating an Object That Always Looks at a Specific Object
Creating an Object That Always Looks at the Player
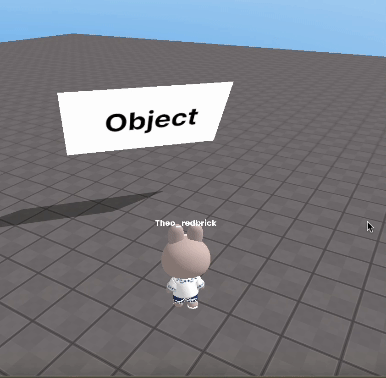
You can easily implement this using the .lookAt()
method.
const obj = WORLD.getObject("object");
function Update() {
obj.lookAt(PLAYER.position.x, PLAYER.position.y, PLAYER.position.z);
}
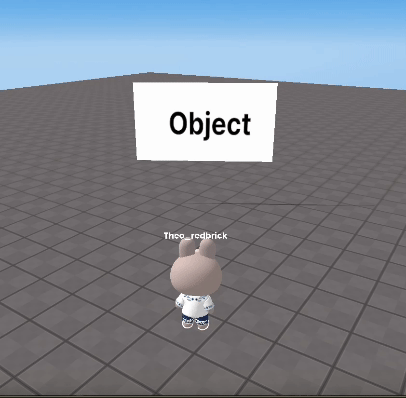
There are two methods.
The first is to use .lookAt()
to make the object look at the player, then set only the x and z rotation values to 0.
The second is to calculate the direction using a vector and rotate only the Y-axis.
const obj = WORLD.getObject("object");
function Update() {
obj.lookAt(PLAYER.position.x, PLAYER.position.y, PLAYER.position.z);
// Set only the x and z rotation values to 0
obj.quaternion.x = 0;
obj.quaternion.z = 0;
}
const obj = WORLD.getObject("object");
function Update() {
const direction = new THREE.Vector3().subVectors(PLAYER.position, obj.position); // Calculate direction
obj.rotation.y = Math.atan2(direction.x, direction.z); // Rotate object only on Y-axis
}
Creating an Object That Always Looks at the Camera
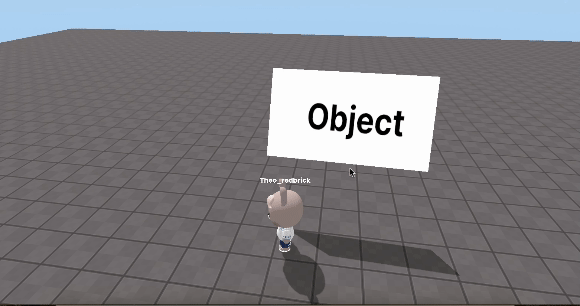
You can easily implement this using the .lookAt()
method.
const obj = WORLD.getObject("object");
const camera = WORLD.getObject("MainCamera");
function Update() {
obj.lookAt(camera.position.x, camera.position.y, camera.position.z);
}
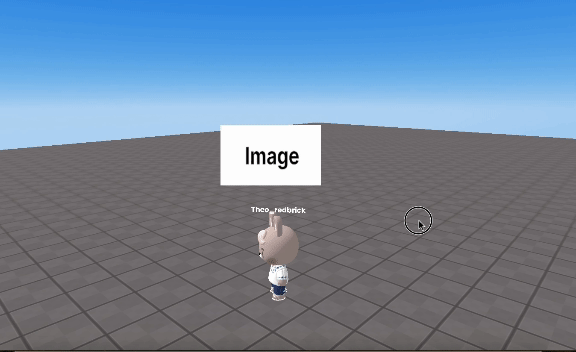
If the object you want to look at the camera is an image, you can also implement this using Sprite
provided by Three.js.
const image = WORLD.getObject("image"); // Retrieve the image object added to the scene
const sprite = new THREE.Sprite(new THREE.SpriteMaterial({map: image.material.map})); // Create a Sprite object with the image
sprite.position.set(10, 5, 10); // Set the position of the sprite
sprite.scale.set(5, 3, 1); // Set the scale of the sprite
WORLD.add(sprite); // Add to the world for rendering