Create GUI Animation
This tutorial demonstrates how to create GUI animations using TWEEN.
GUI animations can be created by adjusting the GUI’s Offset.
We will use TWEEN’s .easing()
and .yoyo()
functions.
Adding an Image to the GUI for Animation
Add the image you want to animate to the GUI.
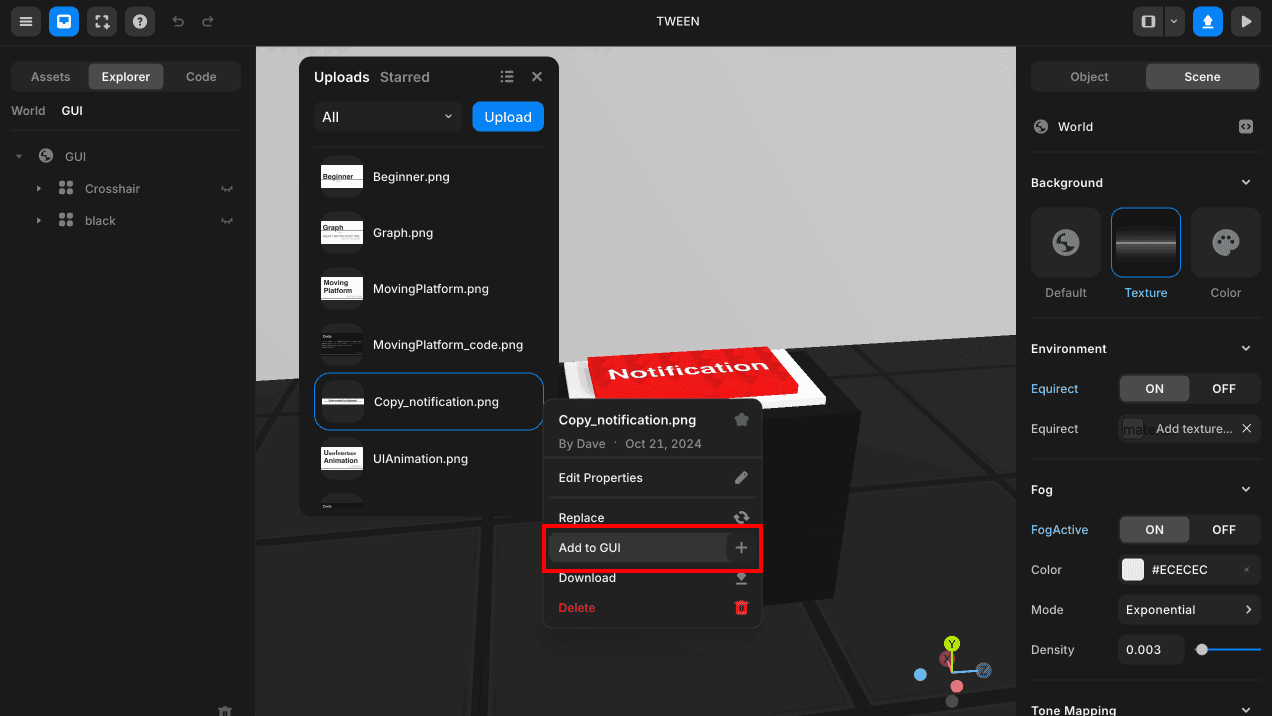
Basic Setup for the Image GUI
a. Set the size of the image.
Make sure to turn on Maintain AspectRatio to keep the ratio, change the unit to percent (%), and then adjust the size.
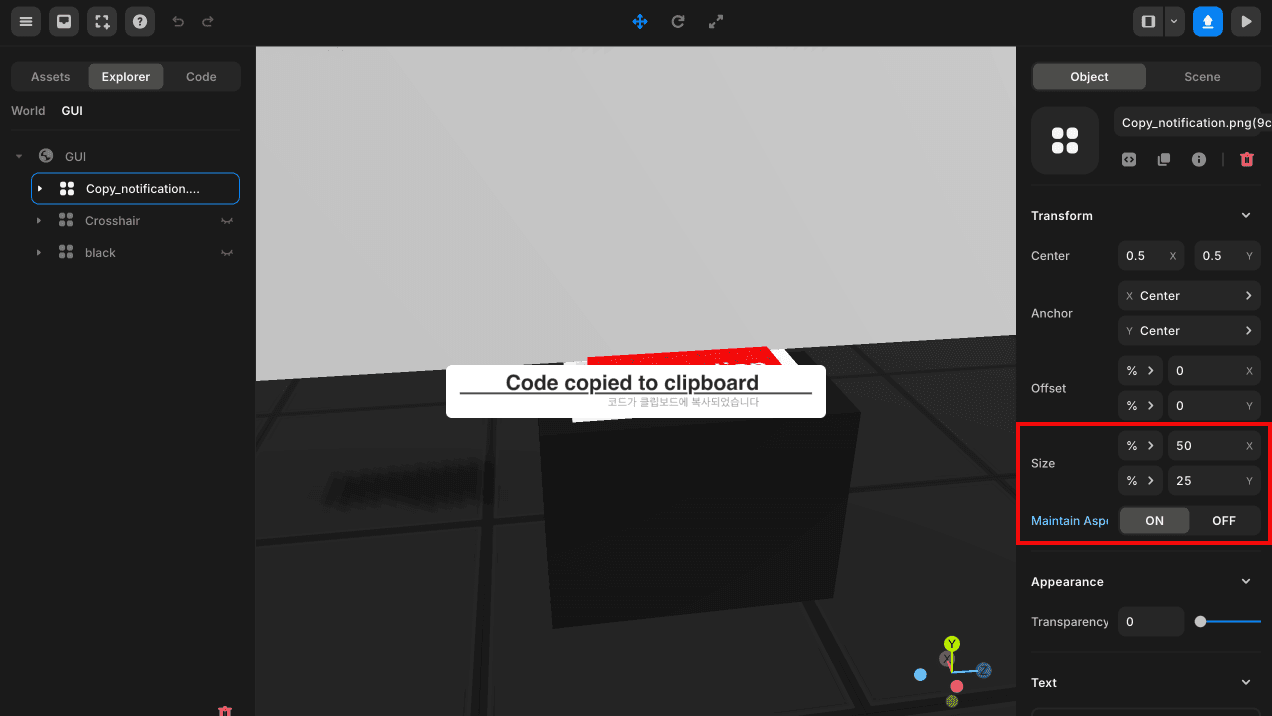
b. Set the starting position of the image.
Use the Anchor to define the reference point for the image and adjust the Offset.
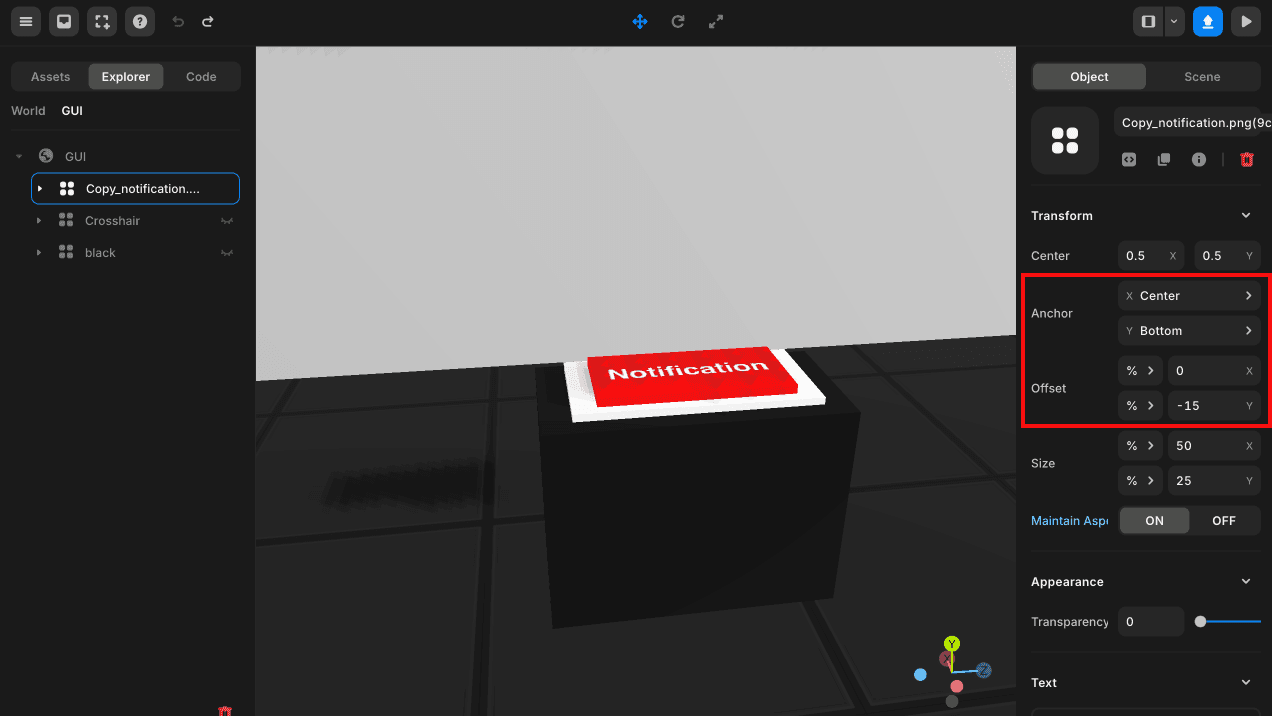
To create a responsive GUI, you must change the unit from px to % (percent).
Additionally, you can toggle Maintain AspectRatio ON/OFF based on whether you want to maintain the ratio.
Writing TWEEN Script for GUI Animation
Add the object (client) script to the image GUI. Use TWEEN to change the image’s Offset.
The offset object can be simply viewed as { x : { value : Number }, y : { value : Number } }
. If you want to change the value along only one axis, you should use ui.offset.y
as the starting object for TWEEN.
Since we are using .yoyo()
, the GUI animation time will be double the duration
in tween.to(object, duration)
.
You can change the object’s movement (interpolation equation) using .easing()
.
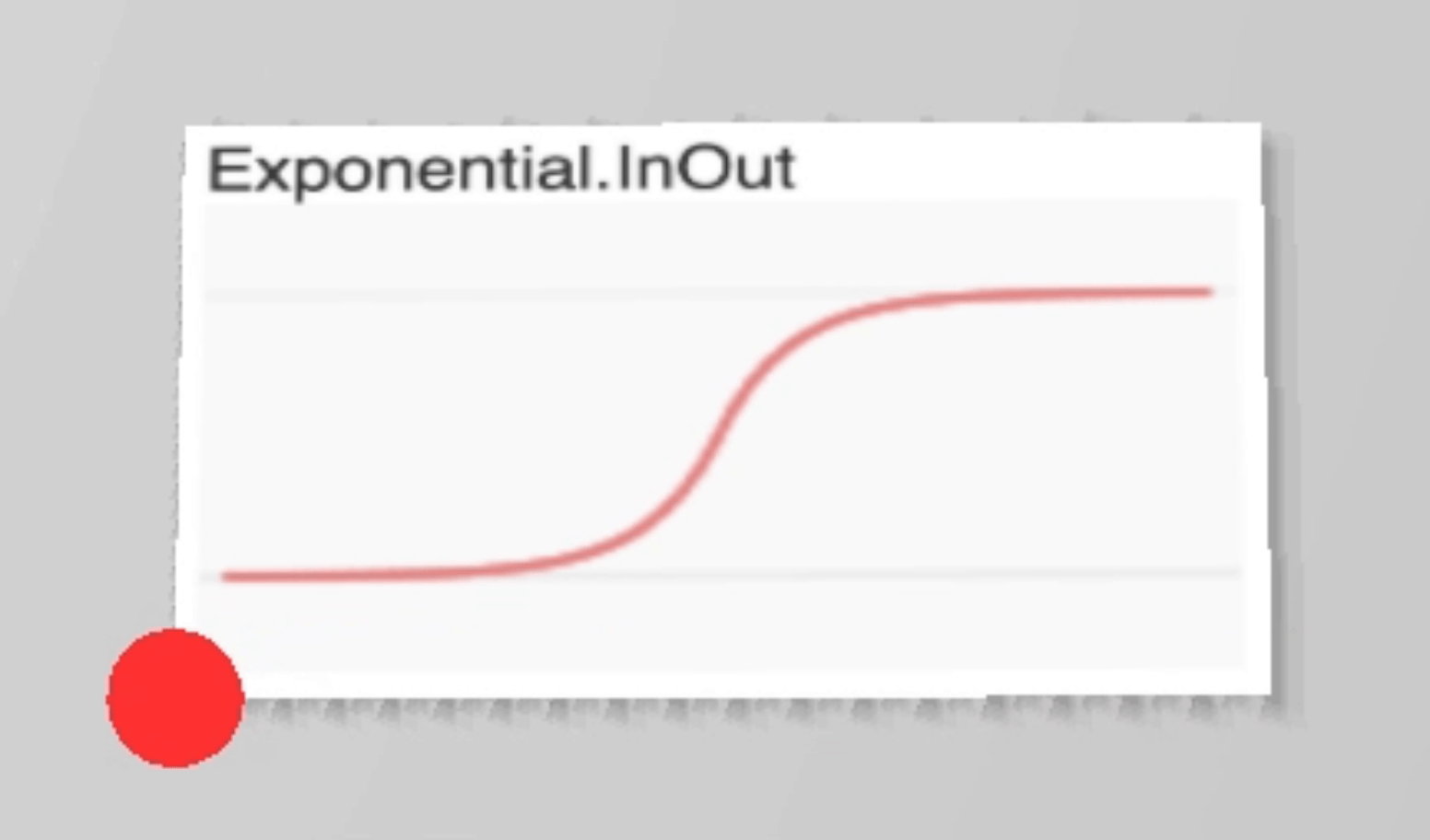
TWEEN.Easing.Exponential.InOut
const ui = GUI.getObject('Copy_notification.png(9cf)');
const tween = new TWEEN.Tween(ui.offset.y);
// Adjust the GUI animation time, the default is 1000ms
tween.to({ value : 20 });
// You can change the movement (interpolation equation) using .easing().
tween.easing(TWEEN.Easing.Exponential.InOut)
.yoyo(true).repeat(1);
The default transition time for TWEEN is 1000 milliseconds, or 1 second.
Executing TWEEN When a Specific Event Occurs
Below is an example of executing TWEEN when the “C” key is pressed.
When the desired event occurs to execute this TWEEN, you can use tween.start()
.
// ...
function OnKeyDown(event) {
if(event.code === 'KeyC') {
if(tween.isPlaying()) {
tween.stop();
ui.offset.y.value = -15; // Initial position
}
tween.start();
}
}
If the GUI animation restarts before it finishes, stop it and reset the position value to ensure the animation starts from the initial position.