Create a Boomerang
This tutorial demonstrates how to create a boomerang using TWEEN.
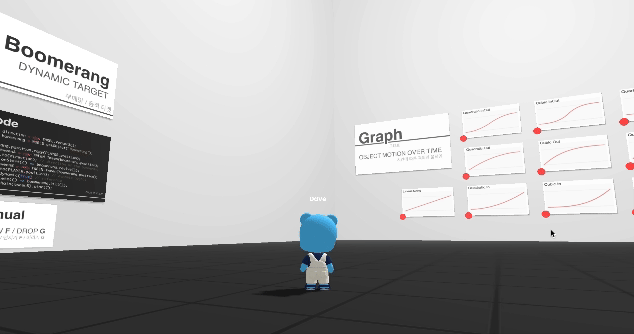
A boomerang can be created by adjusting the position of the boomerang object.
We will use TWEEN’s .chain()
and .dynamic()
methods.
Adding the Boomerang Object
Add a torus mesh.
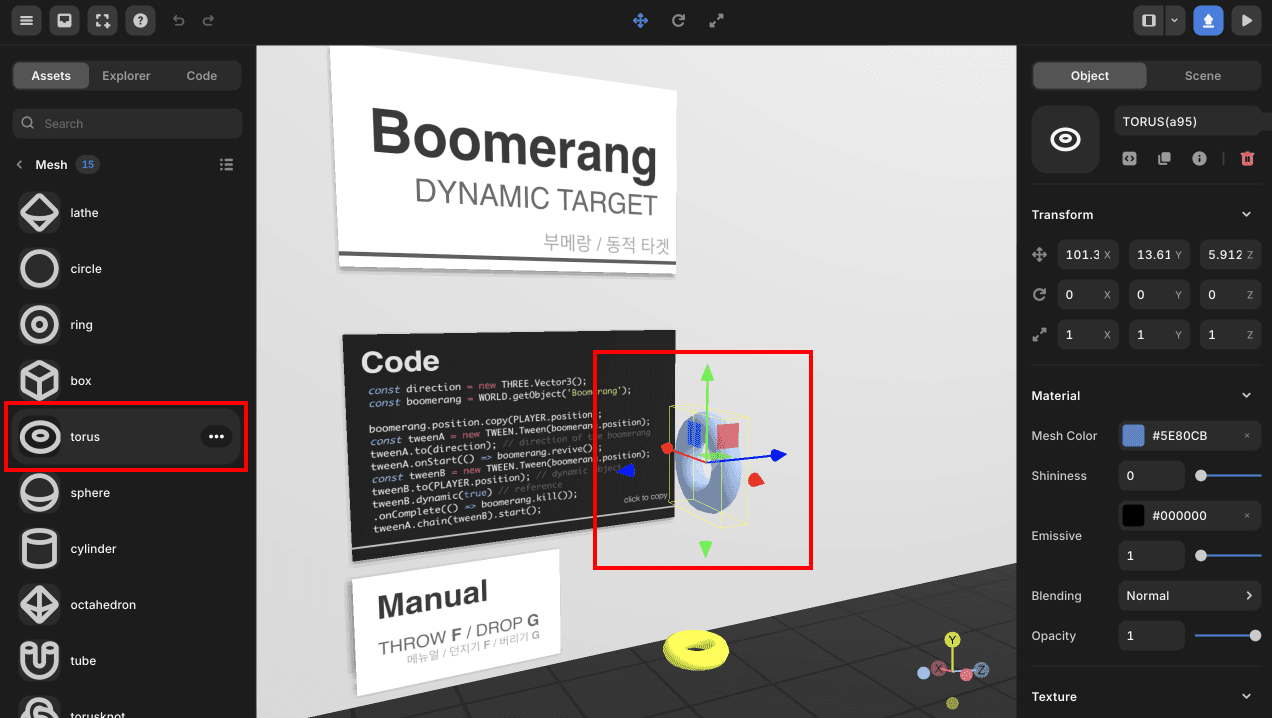
Basic Setup for the Boomerang
a. Set the object’s name to Boomerang.
b. Change the object’s rotation values.
c. Change the object’s color.
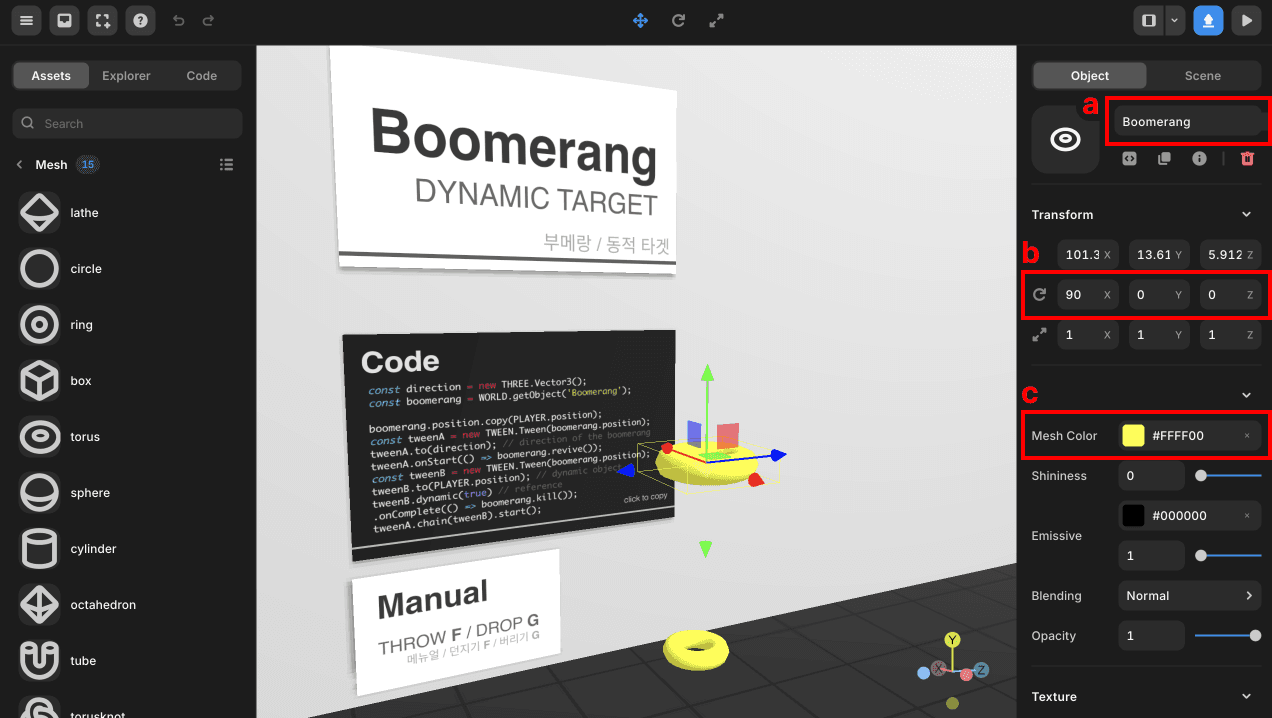
Writing the TWEEN Script for the Boomerang
a. Write a script to set the target position of the boomerang.
Use the camera’s direction (forward) to set the target position. Since the target position needs to continuously change based on the camera’s movement, place this in the Update function.
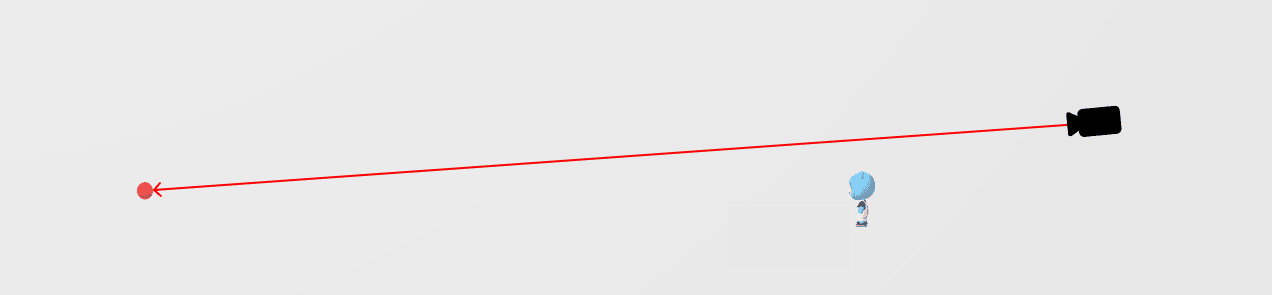
const camera = WORLD.getObject('MainCamera');
const direction = new THREE.Vector3();
function Update(dt) {
camera.getWorldDirection(direction);
direction.multiplyScalar(60);
direction.add(camera.position);
}
b. Write the TWEEN script for the boomerang.
The boomerang TWEEN consists of two TWEENs connected by .chain()
.
The first TWEEN moves to the target position, and the second TWEEN returns it to the player.
Since the player is a moving object, use .dynamic()
to allow the target object to be referenced.
Both TWEENs use the default transition time, so the total transition time will be 2 seconds.
// ...
const boomerang = WORLD.getObject('Boomerang');
let isPlaying = false;
function boomerangStart() {
boomerang.position.copy(PLAYER.position);
const tweenA = new TWEEN.Tween(boomerang.position);
// Adjust the time it takes for the boomerang to fly, the default is 1000ms
tweenA.to(direction);
tweenA.onStart(() => {
isPlaying = true;
boomerang.revive();
});
const tweenB = new TWEEN.Tween(boomerang.position);
tweenB.to(PLAYER.position);
tweenB.dynamic(true)
.onComplete(() => {
isPlaying = false;
boomerang.kill();
});
tweenA.chain(tweenB).start();
}
The default transition time for TWEEN is 1000 milliseconds, or 1 second.
Since the two TWEENs are connected by .chain()
, a separate variable is needed to track whether the current TWEEN is playing.
Use the .onStart()
of the first TWEEN and the .onComplete()
of the second TWEEN to update this variable.
Executing TWEEN When a Specific Key is Pressed
Execute the TWEEN when the ‘F’ key is pressed. You can change line 4 to your desired key.
// ...
function OnKeyDown(event) {
if(event.code === 'KeyF') boomerangStart();
}