Flow control
Hereâs a guide on the code execution flow in Redbrick Studio.
What is Code Flow Control?
Consider the following code:
function func1 () {
console.log("Execute the first function.");
};
function func2 () {
console.log("Execute the second function.");
};
console.log("Hi Redbrick!");
func1();
func2();
When you run the above code, the result appears as follows.
> Hi Redbrick!
> Execute the first function.
> Execute the second function.
You can observe that the code runs in the order from top to bottom.
This sequence of executing commands in a program is what we refer to as the code execution flow.
Flow control involves managing this sequence of execution.
Types of Flow control
In Redbrick Studio, the code execution flow can be broadly divided into two categories: external flow from scripts and internal flow within scripts.
internal flow within scripts
console.log("Hi Redbrick!");
function Start() {
console.log("I like Redbrick");
}
function Update() {
console.log("I like coding");
}
console.log("welcome to Redbrick land!");
When you run the above code, the result appears as follows.
> Hi Redbrick!
> welcome to Redbrick land!
> I like Redbrick
> I like coding
> I like coding
> I like coding
> I like coding
> I like coding
...
When executing code within the script, all code except for Start and Update functions runs first.
After the Start function executes, the Update function runs last.
In addition, excluding event functions like onClick()
, the code executes only once when the script runs initially.
However, the Update function runs every frame.
Therefore, unless a separate termination condition is provided, the message âI like codingâ will continue to be output continuously until the game ends.
external flow from scripts
Figure 1 - script window
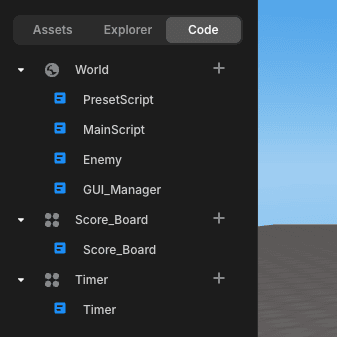
As you saw in the above code, in the Redbrick Engine, code is executed in the order from top to bottom.
This applies to scripts as well.
The scripts visible in the above picture are executed in the following order:
Figure 2 - Execution order of the script
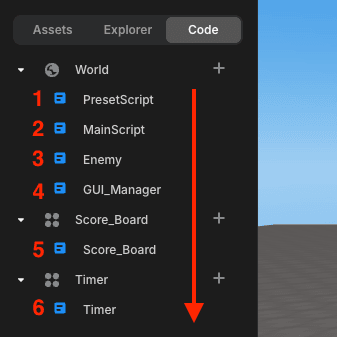
The script âPresetScriptâ at the top is executed first, followed by the scripts below in sequence, ending with âTimerâ being executed last.
The internal code of âPresetScriptâ and âMainScriptâ is as follows:
console.log(GLOBAL.variable);
GLOBAL.variable = "Hello World!";
In âPresetScriptâ, variables declared in âMainScriptâ are referenced.
However, since âMainScriptâ is positioned below âPresetScriptâ, when the console.log(GLOBAL.variable)
code is executed, it attempts to reference GLOBAL.variable
which has not yet been declared.
Therefore, the result is as follows:
> undefined
When attempting to reference a variable GLOBAL.variable
that has not yet been declared, the result indicates that GLOBAL.variable
remains undefined.
Combining Internal and External Script Flows
In Figure 2 above, when combining the flow of code both internally and externally, it unfolds as follows:
> Execution of general code in PresetScript
> Execution of general code in MainScript
> Execution of general code in Enemy
> Execution of general code in GUI_Manager
> Execution of general code in Score_Board
> Execution of general code in Timer
> Execution of Start function in PresetScript
> Execution of Start function in MainScript
> Execution of Start function in Enemy
> Execution of Start function in GUI_Manager
> Execution of Start function in Score_Board
> Execution of Start function in Timer
> Execution of Update function in PresetScript
> Execution of Update function in MainScript
> Execution of Update function in Enemy
> Execution of Update function in GUI_Manager
> Execution of Update function in Score_Board
> Execution of Update function in Timer
Similarly, the scripts iterate from top to bottom, executing each scriptâs general code (excluding Start and Update functions) first.
Afterward, it goes back to the top and executes the Start functions of each script in sequence.
Then, it iterates upwards again to execute the Update functions in order.